728x90
반응형
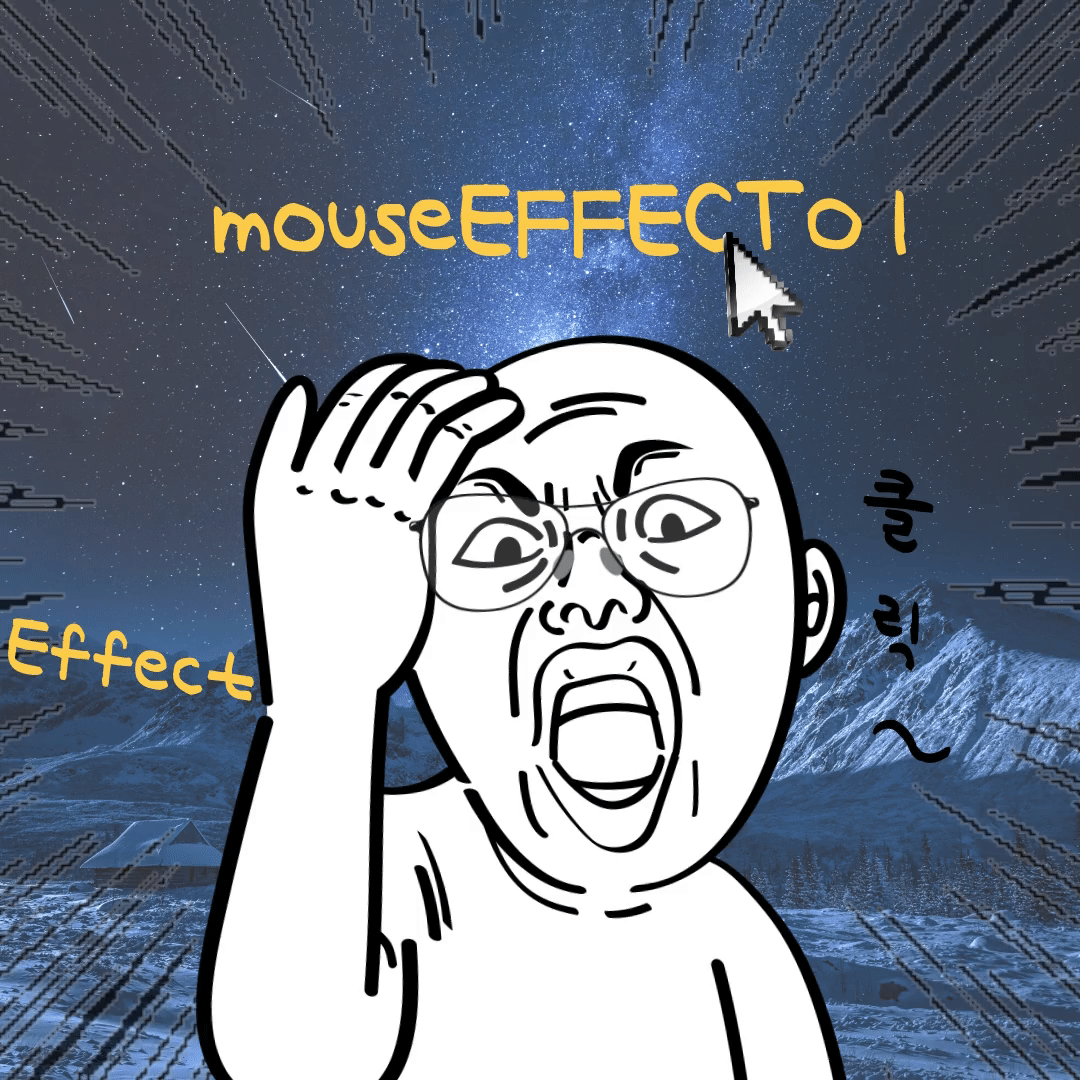
마우스 유형01
마우스 이펙트는 마우스 커서 해당위치에 커서대신 다양한 효과를 주거나 요소에 위치에서 다양한 변화를 주는 효과입니다.
CSS
css로 커서의 모양과 해당 요소 위에서 애니메이션을 주었습니다.
/* mouseType */
.mouse__wrap {
width: 100%;
height: 100vh;
display: flex;
align-items: center;
flex-direction: column;
justify-content: center;
color: #fff;
overflow: hidden;
cursor: none;
}
.mouse__wrap p {
font-size: 2vw;
line-height: 2;
font-weight: 300;
}
.mouse__wrap p span {
border-bottom: 0.15vw dashed rgb(0, 137, 250);
color: rgb(0, 137, 250);
}
.mouse__wrap p:last-child {
font-size: 3vw;
font-weight: 400;
}
@media (max-width: 800px) {
.mouse__wrap p {
padding: 0 20px;
font-size: 20px;
line-height: 1.5;
text-align: center;
margin-bottom: 10px;
}
.mouse__wrap p:last-child {
font-size: 40px;
line-height: 1.5;
text-align: center;
word-break: keep-all;
/* 단어로 줄바꿈 */
}
}
.mouse__cursor {
position: absolute;
left: 0;
top: 0;
width: 50px;
height: 50px;
border-radius: 50%;
border: 3px solid #fff;
background-color: rgba(255, 255, 255, 0.1);
/* 커서 영역 이벤트 발생 x */
user-select: none;
pointer-events: none;
transition: background-color 0.3s, border-color 0.3s, transform 0.6s, border-radius 0.5s;
}
/* 스타일에 따라 마우스 이펙트가 변하도록 */
.mouse__cursor.style1 {
background-color: rgba(55, 0, 255, 0.479);
border-color: rgb(55, 0, 255);
transform: skew(40deg);
}
.mouse__cursor.style2 {
background-color: #f5f5;
border-color: #f5f5;
transform: scale(2) rotateY(720deg);
}
.mouse__cursor.style3 {
background-color: rgba(4, 255, 45, 0.459);
border-color: rgba(4, 255, 45);
transform: scale(1.5) rotateX(360deg);
}
.mouse__cursor.style4 {
background-color: rgba(0, 204, 255, 0.333);
border-color: rgba(0, 204, 255);
transform: scale(10);
}
.mouse__cursor.style5 {
background-color: rgba(255, 0, 0, 0.333);
border-color: rgba(255, 0, 0);
border-radius: 0;
}
.mouse__cursor.style6 {
background-color: rgba(208, 255, 0, 0.333);
border-color: rgba(208, 255, 0);
transform: scale(10);
border-radius: 0;
}
.mouse__info {
position: absolute;
left: 20px;
bottom: 10px;
font-size: 14px;
line-height: 1.6;
color: #fff;
}
HTML
html은 명언에 글자마다 다른 스타일의 클래스를 부여하여 다른 애니메이션을 줄 수 있도록 만들었습니다.
<section id="mouseType">
<div class="mouse__cursor"></div>
<div class="mouse__wrap">
<p>The <span class="style1">less</span> their <span class="style2">ability</span>, the more their <span
class="style3">conceit</span></p>
<p><span class="style4">능력</span>이 <span class="style5">부족</span>할 수록 <span class="style6">자만심</span>이 더
강하다</p>
</div>
</section>
Javascript
마우스 커서의 위치를 mousemove를 사용하여 구하도록 합니다.
명언에 각 글자 부분에 style을 설정하여 각각 transition을 설정하여 다른 애니메이션이 생기도록 합니다.
getAttribute 메서드를 사용하여 span 요소의 클래스에 마우스가 나갈때와 들어갈때 class를 설정합니다.
// 마우스 따라다니는 효과
window.addEventListener("mousemove", (event) => {
document.querySelector(".clientX").innerText = event.clientX;
document.querySelector(".clientY").innerText = event.clientY;
document.querySelector(".offsetX").innerText = event.offsetX;
document.querySelector(".offsetY").innerText = event.offsetY;
document.querySelector(".pageX").innerText = event.pageX;
document.querySelector(".pageY").innerText = event.pageY;
document.querySelector(".screenX").innerText = event.screenX;
document.querySelector(".screenY").innerText = event.screenY;
});
const cursor = document.querySelector(".mouse__cursor"); //마우스 커서
const color = document.querySelectorAll(".mouse__wrap span"); // 마우스가 애니메이션 생기는 위치
window.addEventListener("mousemove", (e) => { //마우스가 움직일 때 따라다닐 수 있게 위치 값을 구함
cursor.style.left = e.clientX - 25 + "px";
cursor.style.top = e.clientY - 25 + "px"; // 마우스 커서가 정중앙에 오도록 25를 빼준다.
});
// for문
// for (let i = 1; i < 7; i++) {
// const cursor = document.querySelector(".mouse__cursor");
// document.querySelector(".style" + i).addEventListener("mouseover", () => {
// cursor.classList.add("style" + i);
// });
// document.querySelector(".style" + i).addEventListener("mouseout", () => {
// cursor.classList.remove("style" + i);
// });
// }
// forEach문
// document.querySelectorAll(".mouse__wrap span").forEach((span, num) => {
// span.addEventListener("mouseover", () => {
// cursor.classList.add("style" + (num + 1));
// });
// span.addEventListener("mouseout", () => {
// cursor.classList.remove("style" + (num + 1));
// });
// });
// getAttribute 각 Element요소의 지정된 속성 값을 반환합니다.
document.querySelectorAll(".mouse__wrap span").forEach((span, num) => { //class를 추가하여 애니메이션이 생기도록 함
let attr = span.getAttribute("class")
span.addEventListener("mouseover", () => {
cursor.classList.add(attr);
});
span.addEventListener("mouseout", () => {
cursor.classList.remove(attr);
});
});
// document.querySelector(".style2").addEventListener("mouseover", () => {
// cursor.classList.add("style2");
// });
// document.querySelector(".style2").addEventListener("mouseout", () => {
// cursor.classList.remove("style2");
// });
// document.querySelector(".style3").addEventListener("mouseover", () => {
// cursor.classList.add("style3");
// });
// document.querySelector(".style3").addEventListener("mouseout", () => {
// cursor.classList.remove("style3");
// });
// document.querySelector(".style4").addEventListener("mouseover", () => {
// cursor.classList.add("style4");
// });
// document.querySelector(".style4").addEventListener("mouseout", () => {
// cursor.classList.remove("style4");
// });
// document.querySelector(".style5").addEventListener("mouseover", () => {
// cursor.classList.add("style5");
// });
// document.querySelector(".style5").addEventListener("mouseout", () => {
// cursor.classList.remove("style5");
// });
// document.querySelector(".style6").addEventListener("mouseover", () => {
// cursor.classList.add("style6");
// });
// document.querySelector(".style6").addEventListener("mouseout", () => {
// cursor.classList.remove("style6");
// });
결과
반응형
'Effect' 카테고리의 다른 글
마우스 이펙트03 : 조명 효과 (2) | 2022.09.23 |
---|---|
마우스 이펙트02 - 마우스 따라다니기(GSAP) (1) | 2022.09.22 |
패럴랙스 - 이질감 효과 (5) | 2022.09.20 |
패럴렉스 - 연속적으로 나타나기 (3) | 2022.09.18 |
패럴랙스 - 사이드 메뉴 (3) | 2022.09.09 |